Integration Steps
A comprehensive guide to integrating your iOS app with AppVirality’s iOS SDK.
Kindly use the same APPKEY for all platforms
If you have registered your Android Application already and want to run the same referral campaign on iOS App as well, do not register the app on AppVirality again, separately. Use the same App Key obtained from the initial registration.
Please keep in mind that the AppVirality iOS SDK is supported for iPhone simulator 5S (or higher), and iPhone 4s device (and higher). AppVirality iOS SDK supports iOS V6.0 and above.
Please contact us if you need support for lower versions.
Additionally, going forward, we shall be denoting the invitation or referral sender as ‘Referrer‘ and the receiveror beneficiary as ‘Friend‘
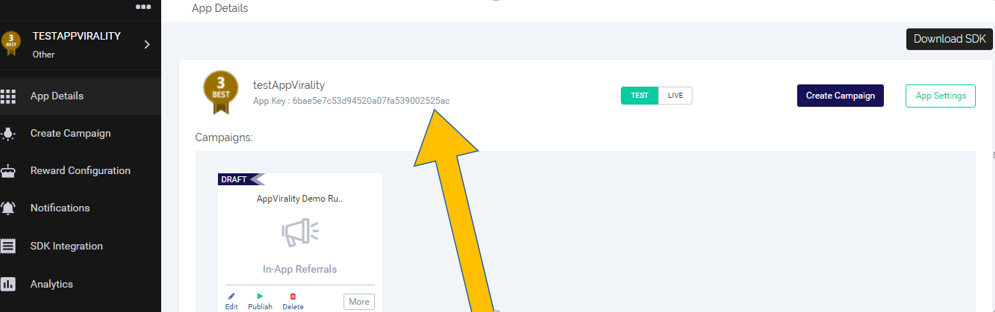
Download the latest AppVirality iOS SDK from here.
Drop the “includes” folder and “libAppVirality.a” file into your project root.
Import “AppVirality.h” the header file
#import "AppVirality.h"
Initializing the AppVirality SDK has to be done at the very beginning of your App i.e. at –application:didfinishlaunchingwithoptions: method in AppDelegate file.
(BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { NSSetUncaughtExceptionHandler(&onUncaughtException); // Override point for customization after application launch. // Enable cookie based attribution to achieve 100% attribution accuracy [AppVirality attributeUserBasedonCookie:AppVirality_AppKey OnCompletion:^(BOOL success, NSError *error) { NSDictionary * userDetails = nil; //if your App has login/logout and would like to allow multiple users to use single device, uncomment below lines [AppVirality enableInitWithEmail]; userDetails = @{@"EmailId":@"USER-EMAIL-ID",@"ReferrerCode":@"REFERRER-CODE",@"isExistingUser":@"false",@"country":@"USER-COUNTRY"}; // Init AppVirality SDK [AppVirality initWithApiKey:AppVirality_AppKey WithParams:userDetails OnCompletion:^(NSDictionary *referrerDetails,NSError*error) { //NSLog(@"user key %@",[[NSUserDefaults standardUserDefaults] valueForKey:@"userkey"]); //NSLog(@"User has Referrer %@", referrerDetails); }]; }]; return YES; }
Replace “YOUR_APP_KEY” in the above code block, with your App key from your app’s dashboard. You can get your App key from AppVirality Dashboard → App Details page.
This is required if you want to show the welcome screen on the first App launch. You can get the referrer details by calling the below code block.
[AppVirality getReferrerDetails:^(NSDictionary *referrerDetails, NSError *error) { NSLog(@"ref details %@",referrerDetails); }];
The following callback method will return the referral campaign details. Use the campaign details to show the referral screen to the App users.
campaignDetails includes the list of social actions configured on AppVirality Dashboard. Please use the array with name socialactions to get the social share messages and unique share links for each social action.
[AppVirality getGrowthHack:growthHack completion:^(NSDictionary *campaignDetails,NSError*error) { NSLog(@"growth hack details %@",campaignDetails); }];
growthHack – Type of growth hack i.e. to get referral campaign use “GrowthHackTypeWordOfMouth”
Call the below method after successful completion of the social action. i.e after sharing on social media. This records the user social action.
[AppVirality recordSocialActionForGrowthHack:GrowthHackTypeWordOfMouth WithParams:@{@"shareMessage":[[campaignDetails valueForKeyPath:@"socialactions.shareMessage"]firstObject],@"socialActionId":[[campaignDetails valueForKeyPath:@"socialactions.socialActionId"]firstObject],@"shortcode":[campaignDetails valueForKey:@"shortcode"]} completion:^(BOOL success,NSError*error) { NSLog(@"social sucess %d",success); }];
Before recording any conversion event, please make sure to update the user details(at least EmailID) to AppVirality as noted/described in STEP 9. User details are critical to process rewards for the end user.
[AppVirality saveConversionEvent:@{@"eventName":@"Transaction",@"transactionUnit":@"Rs",@"transactionValue":@"560",@"extrainfo":@"orderid:78F6YG"} completion:^(NSDictionary *conversionResult,NSError *error) { NSLog(@"conversion result %@",conversionResult); }];
This code block would give you the details about any user’s balance/earnings etc.
[AppVirality getUserBalance:self.growthHack completion:^(NSDictionary *userInfo,NSError*error) { self.userPoints = [(NSArray*)[userInfo valueForKey:@"userpoints"] firstObject]; // List of friends installed through the referral link self.referredusers = [userInfo valueForKey:@"referredusers"]; NSLog(@" user balance %@",userInfo); }];
Please set the user details before calling “saveConversionEvent” in STEP 7 described above.
NSDictionary * userDetails = @{@"EmailId":@"mymail@test.com",@"AppUserName":@"CustomerName",@"ProfileImage":@"http://www.pics.com/profile.png",@"UserIdInstore":@"78903",@"city":@"Pune",@"state":@"Maharashtra",@"country":@"India",@"Phone":@"9876543210",@"isExistingUser":@"false",@"pushDeviceToken":@"YOUR-DEVICE-TOKEN"}; [AppVirality setUserDetails:userDetails Oncompletion:^(BOOL success, NSError *error) { NSLog(@"User Details update Status %d", success); }];
Please call this method when user clicks on Logout in your App. This will clear the current user session to allow new user login to the App
[AppVirality logout];
Set isExistingUser property as TRUE only on first App launch i.e. only once.
On subsequent launches please don’t set this property.
At any point of time, excluding the first time, if you set this property to TRUE all the existing user rewards will be set to Rejected state and also stops reward on future events.
Use this property carefully and with caution!
Integration Complete
Use the Sample Application to see the above callbacks in action.